The Premium Java Programming Certification Bundle
What's Included
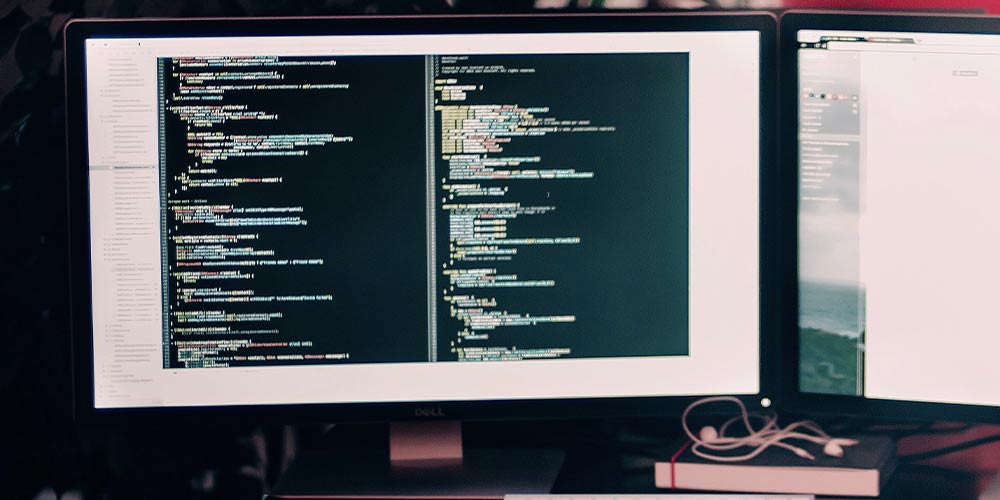
Java: A Complete tutorial from ZERO to JDBC Course
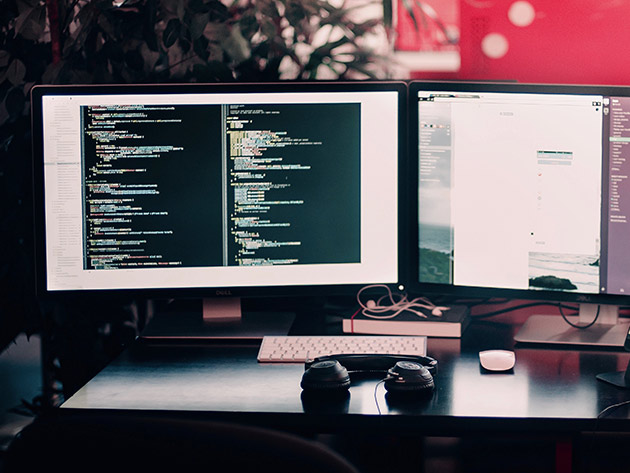
- Experience level required: All levels
- Access 168 lectures & 20 hours of content 24/7
- Length of time users can access this course: Lifetime
Course Curriculum
168 Lessons (20h)
- Your First Program
- Section 0 - Setting up your Java Development KitSection Introduction0:40Setting up your JDK on your Mac8:42Setting up your NetBeans on your Mac8:58Testing your JDK and NetBeans on your Mac7:24Setting up your JDK on your Windows Machine13:06Setting up and Test your NetBeans on your Windows Machine9:44Resource Files
- Section 1 - Your First Java CupYour First Java Program - HelloWorld5:25Commonly Encountered Errors19:57Parts of Your Java Program10:28Resource Files
- Section 2 - Difference between a Class and an ObjectDifference Between a Class and Objects7:10The Person and TestPerson Classes8:03Constructors17:26Methods12:02Encapsulation (aka Data Hiding)18:54The Java API Documentation11:55The import statement13:23Resource Files
- Section 3 - Introducing the use of an Integrated Development Environment (IDE)Integrated Development Environment (IDE)6:06Your First NetBeans Project9:37Comments, Semi-colon, Blocks and Whitespaces18:34The import and the package statements15:54Java Data Types15:27The Scanner object and the nextXxx() Methods9:37Resource Files
- Section 4 - Operators and Control StructuresSection Introduction0:49Data Type Casting, part 14:42Data Type Casting, part 24:07Data Type Casting, part 36:27The Increment and Decrement Operators9:28The Mathematical Operators9:12The Relational Operators3:59The Logical Operators and the Short-Circuit Operators, part 18:19The Logical Operators and the Short-Circuit Operators, part 22:50The Ternary Operator5:28The Ternary Operator Sample Problem - Odd / Even0:22The Ternary Operator Solution - Odd / Even3:21The Assignment and Short-Hand Operators4:38The if-else condition5:47The if-else-if ladder and age problem1:46The age problem solution5:00The nested if7:13The switch-case statement10:32The month to word problem0:33The month to word solution3:30The taxi fare problem1:23The taxi fare solution6:34The while and the do-while loops, part 16:14The while and the do-while loops, part 24:06Infinite Loops5:00Print all numbers from the smaller to the larger number problem0:54Print all numbers from the smaller to the larger number solution4:37The for loop, part 16:26The for loop, part 22:47Print the odd numbers from 1 to 10 problem0:34Print the odd numbers from 1 to 10 solution3:39Nested loops6:58Print the Multiplication Table Problem0:24Print the Multiplication Table Solution2:28The break and continue statements7:11Labelled breaks and labelled continues4:12Resource Files
- Section 5 - The Reference Data TypesThe Reference Data Types9:54User Defined Classes9:55Assigning a Reference Value to a Variable3:44Pass by Value and Local Variable Scopes19:30The String Problem1:00The String Solution4:15The 'this' keyword, part 17:43The 'this' keyword, part 29:43Resource Files
- Section 6 - Arrays and StringsSection Introduction, Arrays and Strings1:17Array Creation and Initialization, part 15:52Array Creation and Initialization, part 28:17Array Limits, the .length attribute5:24Sample Array Problem and Solution8:04The Enhanced for loop7:38Copying of Arrays12:04Command Line Arguments and the parseXxx Methods19:56The Two Dimensional Arrays, aka an Array of Arrays13:04The Multiplication Table Problem0:19The Multiplication Table Solution6:12The Non-Rectangular Arrays6:22The Calendar Problem2:02The Calendar Solution15:57The String, StringBuffer and StringBuilder, part 116:38The String, StringBuffer and StringBuilder, part 25:39The Palindrome Problem0:48The Palindrome Solution4:36Resource Files
- Section 7 - Inheritance and PolymorphismInheritance Concepts8:30Java Access Modifiers3:47Method Overriding8:26The 'super' keyword4:21Polymorphism Concepts7:48The Virtual Method Invocation and Heterogeneous Arrays4:14Polymorphic Arguments, the 'instanceof' operator and obj cast p114:04Polymorphic Arguments, the 'instanceof' operator and obj cast p27:08Overloading Methods10:50Inheritance and Constructors13:07The Object Class and the equals() method12:53The Object Class and the hashCode() method5:19The Object Class and the toString() method4:30The 'static' keyword, part 111:27The 'static' keyword, part 28:39Resource Files
- Section 8 - Other Class FeaturesSection Introduction on Other Class Features0:44The Wrapper Classes9:29The 'final' keyword7:03The 'enum' keyword8:49The 'abstract' keyword9:41Java Interfaces21:23The Interface default methods8:45The Interface static methods3:30Functional Interface and the Lambda Operator12:20Resource Files
- Section 9 - Exceptions and AssertionsSection Introduction on Exceptions and Assertions1:57The Exception and the Error Classes5:34The 'try' and 'catch' blocks8:43The 'finally' block20:18The Exception Hierarchy9:32Multiple Exceptions in a catch block2:14The parameterized try block and the handle-or-declare rule, part 110:04The parameterized try block and the handle-or-declare rule, part 24:10The 'throws' keyword0:56The Rules on Overriding Methods and Exceptions6:16Creating your Own Exception8:45Assertion Checks, the 'assert' keyword5:53Resource Files
- Section 10 - IO and FileIOSection Introduction on IO and File IO0:42How to accept inputs using the Scanner Class, a review part 17:14How to accept inputs using the Scanner Class, a review part 22:13How to accept inputs using the BufferedReader and InputStreamRead10:47How to Format an Output10:32The File Class5:35How to read inputs from a file8:35How to write data to a file17:45Resource Files
- Section 11 - The Collection and Generics FrameworkSection Introduction on the Collection and the Generics Framework0:46The Collection Interface7:07The Set and the List Interfaces6:54The Map Interface5:09The Iterator Interface11:14The Generics Framework9:53Sorting your Set Collection18:35Resource Files
- Section 12 - Building a GUI Based Desktop ApplicationSection Introduction on How to Build a GUI-Based Desktop App2:00The AWT Package, the Component and the Containers3:00The Component and the Containers Examples, part 17:13The Component and the Containers Examples, part 210:01The Layout Managers - The FlowLayout Manager8:40The Layout Managers - The BorderLayout Manager10:36The Layout Managers - The GridLayout Manager6:44Demonstrate How Complex Layout Manager and Nested Layout Manager16:22Events, Event Sources and Event Handlers2:55Implementing the Event Handling Techniques - The Deligation Model8:40Implementing the Event Handling Techniques - Using Listeners9:52Implementing EHT - Using Adapter Classes and Inner Classes8:39Completion of the SimpleCalculator Application15:56Packaging a JAR file for application deployment, part 114:38Packaging a JAR file for application deployment, part 26:42Resource Files
- Section 13 - Introduction to JDBCSection Introduction on Java Database Connectivity (JDBC)0:55How to Create your first DB Schema using NetBeans10:07Steps in using JDBC using the Statement Interface24:42The Statement vs the PreparedStatement Interface10:46Resource Files
Java: A Complete tutorial from ZERO to JDBC Course
LearningWhilePracticing (LWP)
LearningWhilePracticing, founded by Joe Ghalbouni, has one of the most practical approaches when it comes to learning. Their goal is to let you be operational as quickly as possible while at the same grasping all the concepts required. Being able to work on your projects through the software while learning it at the same time, is a source of motivation that will make you go further.
Lawrence Decamora | Oracle Certified Java Programmer
Lawrence Decamora III is a former Java Trainer at Sun Microsystems for 5 years and is currently a full-time Computer Science Instructor at the University of Santo Tomas, Manila, Philippines. He's been using Java for the past 19 years and has earned several Java Related Certifications from Sun, Oracle, and IBM. He has a Bachelor's Degree in Computer Science, a Master's Degree in Educational Management, and currently taking up his degree in Doctor of Education.
Description
Java is one of the most prominent programming languages today due to its power and versatility. In this course, LearnWhilePracticing guides you to be operational right away. This class will help you uncover new skills through practice, instead of going over a boring class where you would not be grasping the concepts. Practices make perfect! Oracle Certified Java Expert, Mr. Lawrence Decamora guides you throughout the whole course. Learn the basics of Java and start writing your own Java program in no time.
- Access 168 lectures & 20 hours of content 24/7
- Learn the essentials of Java
- Understand the difference between a Class & an Object
- Know the use of an Integrated Development Environment (IDE)
- Explore the fundamental concepts of Java
- Build a GUI-based desktop application
- Start working with Java™ database connectivity (JDBC)
"I started this course with no prior knowledge of Java and even coding. It's really amazing what 20 hours of immersing yourself in the Java world can get you. The instructor was very engaging and his demonstrations were very detailed. The pacing of the lessons was on point and each video makes sure you understand each concept before moving on to the next one. I totally recommend this course to anyone that's interested in coding!" – Lian Manongsong
Specs
Important Details
- Length of time users can access this course: lifetime
- Access options: desktop & mobile
- Redemption deadline: redeem your code within 30 days of purchase
- Experience level required: all levels
- Have questions on how digital purchases work? Learn more here
Requirements
- Any device with basic specifications
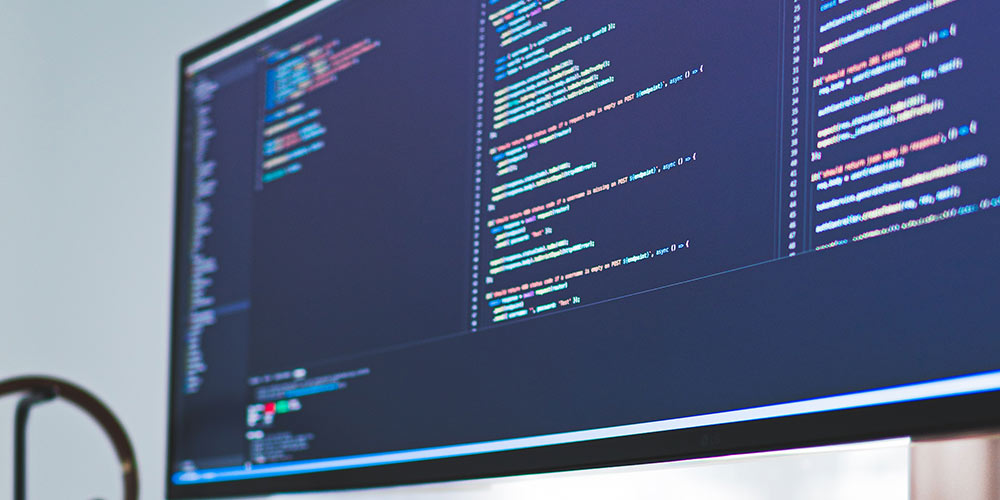
Introduction to Algorithms in Java
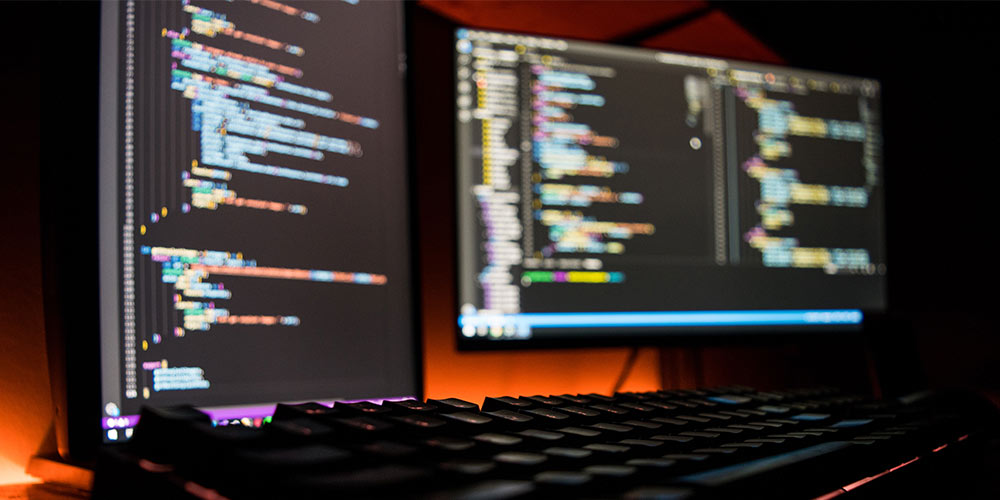
Java Interview Questions: Data Structures & Algorithms
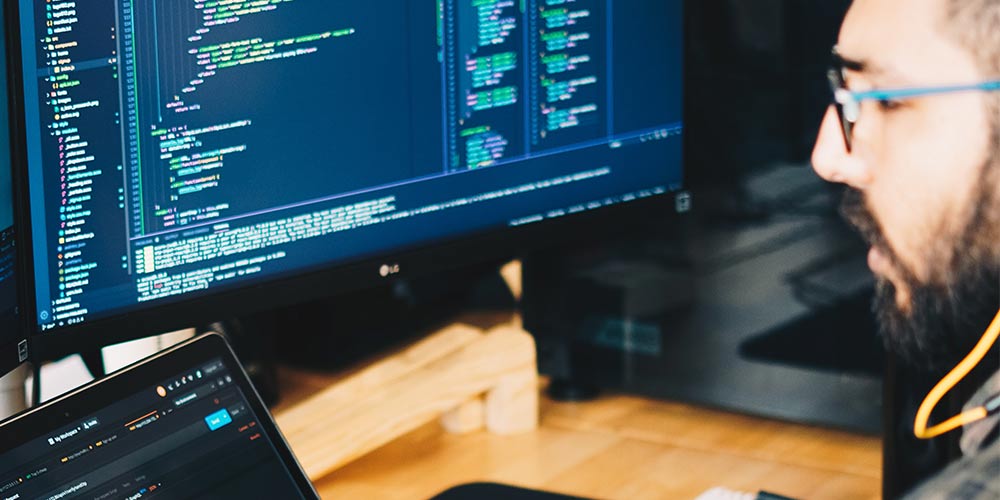
Complete Java Masterclass: Become an Android App Developer
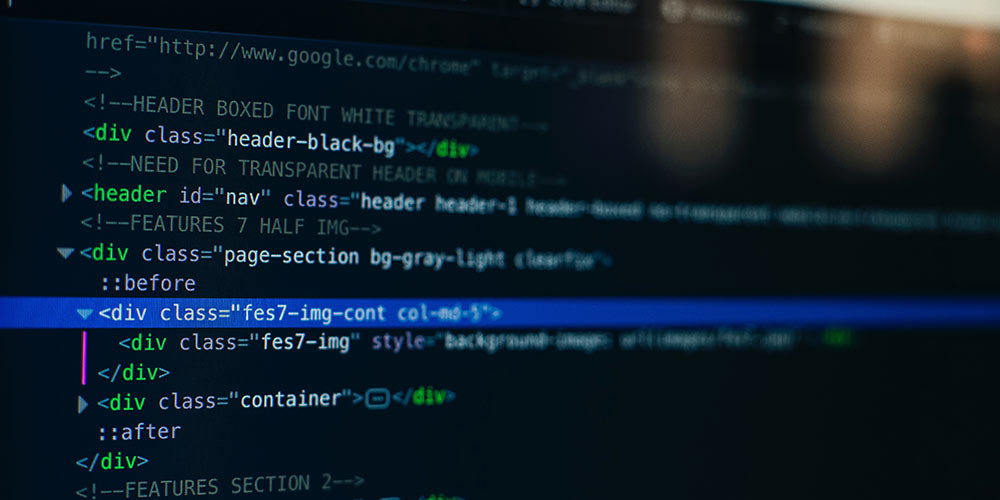
Clean Code with Java examples
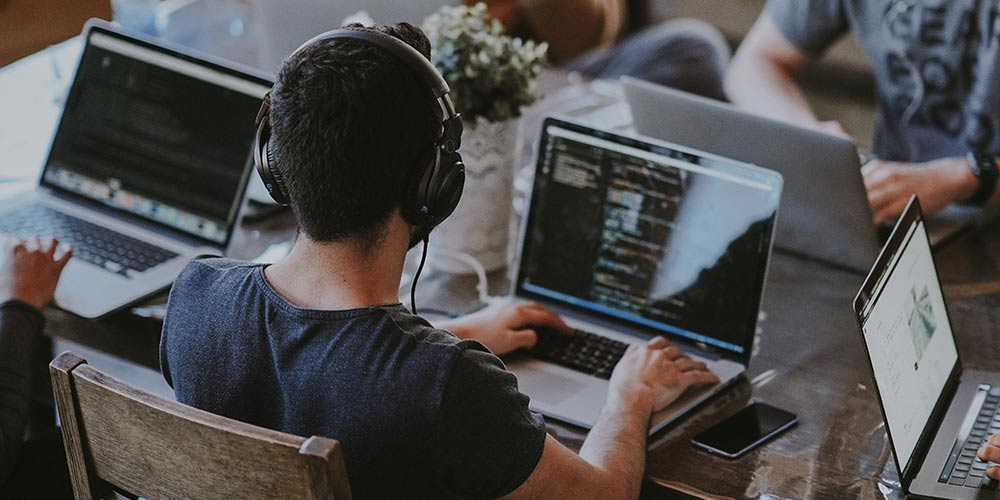
Java Programming: Learn Core Java & Improve Java Skills
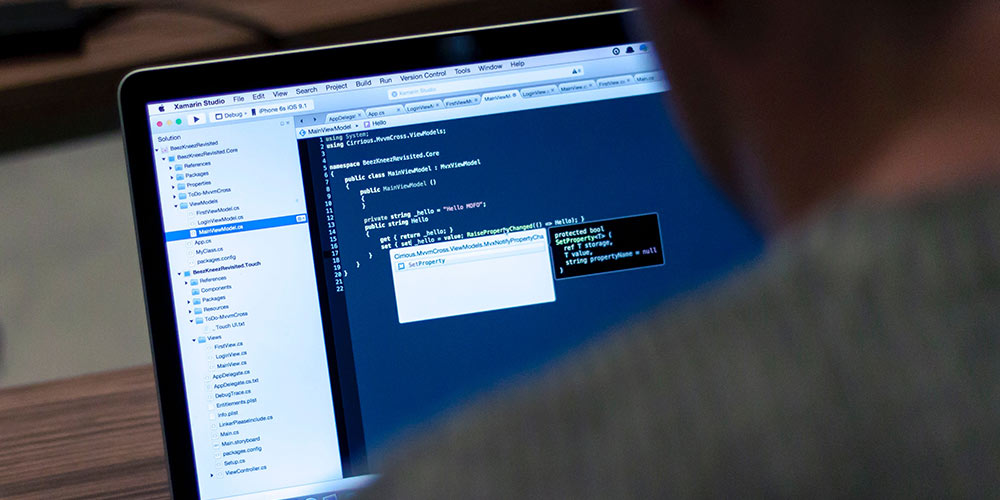
Complete Java Tutorial Step by Step: Become a Programmer
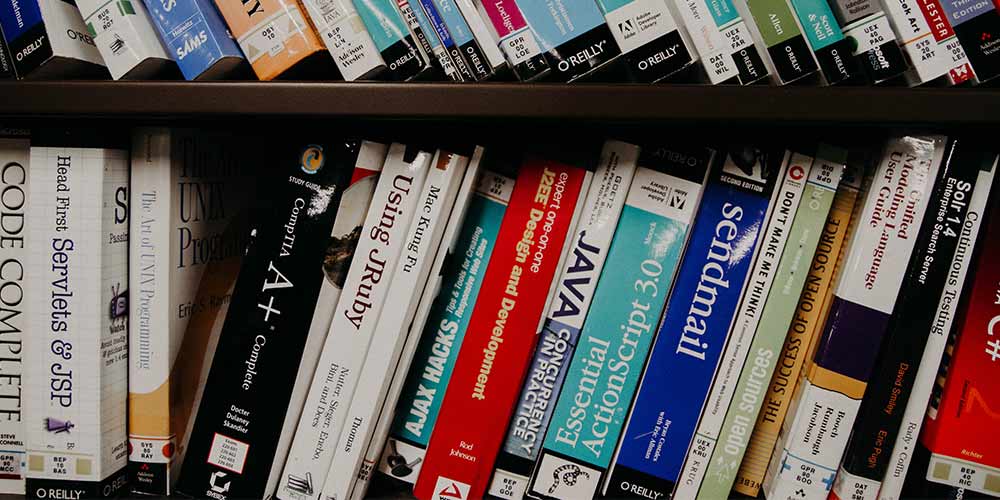
Java Foundations
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.